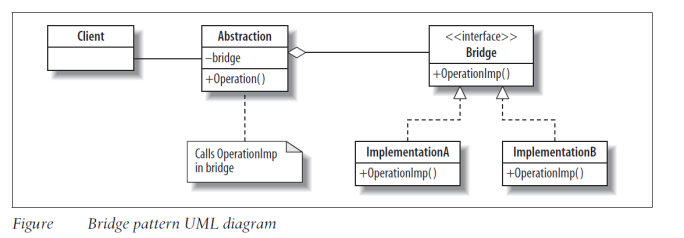
Bridge Pattern
The bridge pattern is a design pattern used in software engineering which is meant to “decouple an abstraction from its implementation so that the two can vary independently”.[1] The bridge uses encapsulation, aggregation, and can use inheritance to separate responsibilities into different classes.
A bridge is a structural pattern that influences the creation of a class hierarchy by decoupling an abstraction from the implementation. In a bridge however, the abstraction and its implementation can vary independently, and it hides the implementation details from the client.
A simple Illustration of Bridge Design Pattern is ‘Remote has a Car object relationship’. This “has-a” relationship bridge is implemented as Bridge Pattern. Two different parts of the code that is changing except the relationship between them then it is bridge between two changing objects.
Inheritance tree of Car with each node representing the different make of Car, is an example of Bridge pattern.
The bridge pattern is useful when both the class as well as what it does vary often. The class itself can be thought of as the implementation and what the class can do as the abstraction.
When a class varies often, the features of object-oriented programming become very useful because changes to a program‘s code can be made easily with minimal prior knowledge about the program with the help of bridge pattern.
The bridge pattern can also be thought of as two layers of abstraction.
The following is the sample code Bridge pattern.
using System; using System.Collections.Generic; using System.Linq; using System.Text; namespace DesignPatterns.Bridge {
public abstract class DataObject
{
public abstract void Register();
public abstract DataObject Copy();
public abstract void Delete();
}
public abstract class Repository
{
public abstract void AddObject(DataObject dataObject);
public abstract void CopyObject(DataObject dataObject);
public abstract void RemoveObject(DataObject dataObject);
public void SaveChanges()
{
Console.WriteLine(“Changes were saved”);
}
}
public class ClientDataObject : DataObject
{
public override void Register()
{
Console.WriteLine(“ClientDataObject was registered”);
}
public override DataObject Copy()
{
Console.WriteLine(“ClientDataObject was copied”);
return new ClientDataObject();
}
public override void Delete()
{
Console.WriteLine(“ClientDataObject was deleted”);
}
}
public class ProductDataObject : DataObject
{
public override void Register()
{
Console.WriteLine(“ProductDataObject was registered”);
}
public override DataObject Copy()
{
Console.WriteLine(“ProductDataObject was copied”);
return new ProductDataObject();
}
public override void Delete()
{
Console.WriteLine(“ProductDataObject was deleted”);
}
}
public class ProductRepository : Repository
{
public override void AddObject(DataObject dataObject)
{
// Do repository specific work
dataObject.Register();
}
public override void CopyObject(DataObject dataObject)
{
// Do repository specific work
dataObject.Copy();
}
public override void RemoveObject(DataObject dataObject)
{
// Do repository specific work
dataObject.Delete();
}
}
}
You should use Bridge pattern whenever you Identify that there are operations that do not always need to be implemented in the same way.
You should implement Bridge pattern when following are the requirements of your application:
• Completely hide implementations from clients.
• Avoid binding an implementation to an abstraction directly.
• Change an implementation without even recompiling an abstraction.
• Combine different parts of a system at runtime.
The next blog I will be explaining about the Builder Pattern.
It is best to participate in a contest for among the best blogs on the web. Ill suggest this web site!